21 January 2025
Python is often hailed as the "Swiss Army Knife" of programming languages. Whether you're just starting your coding journey or you're a seasoned developer, Python offers a versatility and simplicity that’s hard to beat. But, as with any tool, getting the most out of it requires a bit more than just following the tutorials. You need to dig deeper, learn the quirks, and master the nuances.
In this article, we're going to dive into some useful tips and tricks that can help you become a more efficient Python coder. From optimizing your code to writing cleaner, more readable scripts, these insights will help you level up your Python game.
python
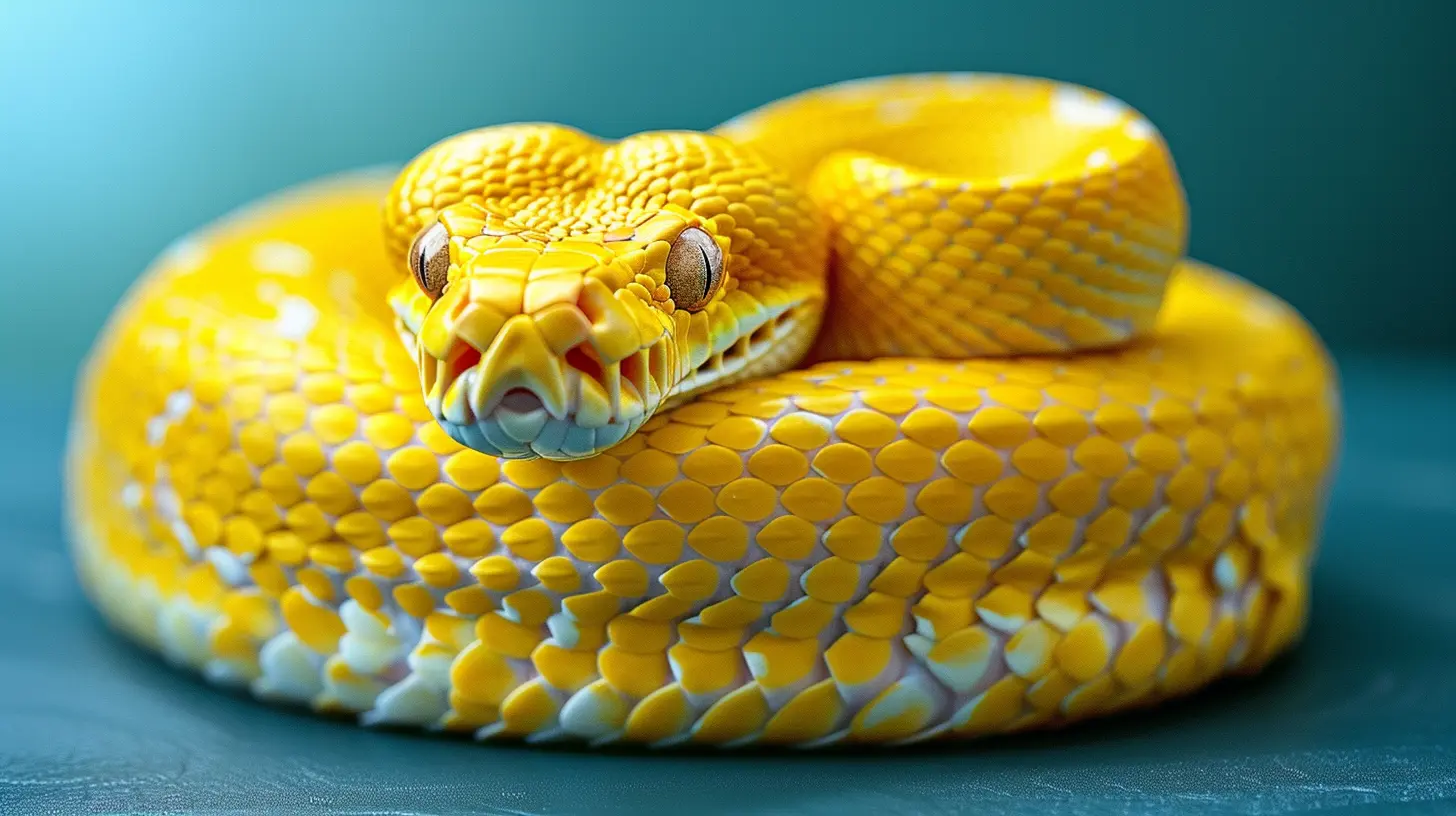
Traditional way
squares = []
for i in range(10):
squares.append(i 2)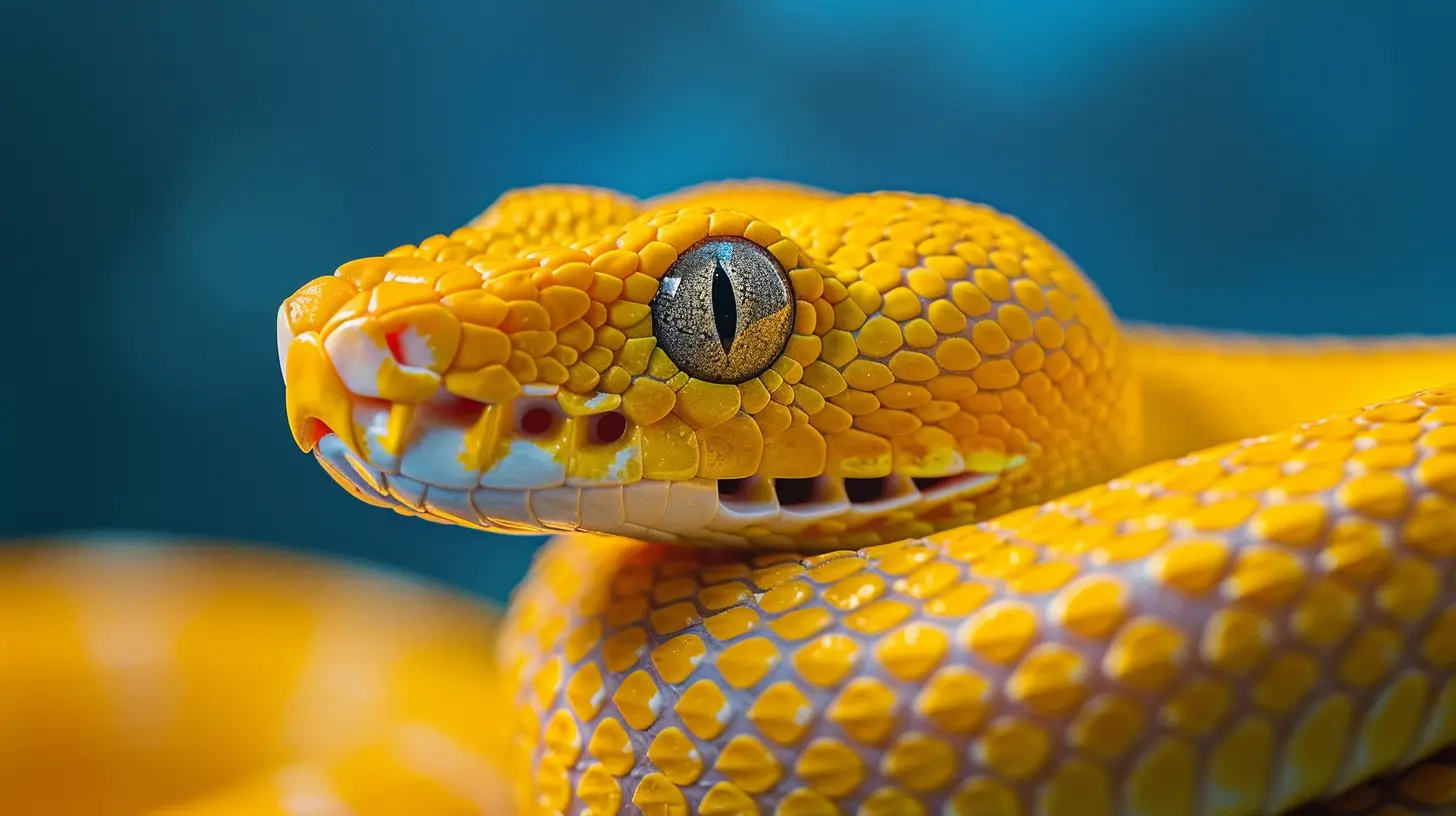
With list comprehension
squares = [i 2 for i in range(10)]
The second version is not only shorter but also easier to read. Once you get the hang of list comprehensions, you'll find they save both time and mental overhead when coding.
python
Using a loop
numbers = [1, 2, 3, 4]
squared = []
for num in numbers:
squared.append(num 2)Using map()
squared = list(map(lambda x: x 2, numbers))
The `map()` function applies the lambda function to each element in the list, making the code shorter and more pythonic.
By leveraging these built-in functions, you can avoid unnecessary loops and make your code more readable and efficient.
python
def my_function(args, *kwargs):
print("Positional arguments:", args)
print("Keyword arguments:", kwargs)my_function(1, 2, 3, name="Alice", age=25)
- `*args`: Collects positional arguments into a tuple.
- `kwargs`: Collects keyword arguments into a dictionary.
This is super handy when you don't know in advance how many arguments a function is going to take. It makes your functions more adaptable and reusable.
python
Traditional way
words = ['apple', 'banana', 'cherry']
for i in range(len(words)):
print(i, words[i])Using enumerate
for i, word in enumerate(words):
print(i, word)
Not only does this make your code cleaner, but it also prevents off-by-one errors that can sneak in when working with indices manually.
python
name = "John"
age = 30Traditional way
print("My name is {} and I am {} years old.".format(name, age))Using f-strings
print(f"My name is {name} and I am {age} years old.")
F-strings are not only more concise, but they are also faster than older methods like `.format()` or `%`-formatting. Plus, they allow you to embed expressions directly into the string, which can be very powerful.
python
from collections import defaultdictTraditional dictionary
normal_dict = {}
normal_dict['a'] = normal_dict.get('a', 0) + 1Using defaultdict
default_dict = defaultdict(int)
default_dict['a'] += 1
This can save you a lot of unnecessary checks and make your code cleaner.
python
Without context manager
file = open('example.txt', 'r')
try:
content = file.read()
finally:
file.close()With context manager
with open('example.txt', 'r') as file:
content = file.read()
Using `with` ensures that the file is always closed, even if an exception occurs. This is not only cleaner but also reduces the risk of resource leaks.
python
List comprehension (stores all numbers in memory)
squares = [i 2 for i in range(1000000)]Generator expression (produces numbers one-by-one)
squares_gen = (i 2 for i in range(1000000))
Generators are especially useful when you're dealing with streams of data or large files. They allow you to process items as they come, which is both faster and more efficient in terms of memory usage.
bash
python -m cProfile my_script.py
`cProfile` will give you a detailed report of how long each function takes to run, allowing you to focus your optimization efforts where they matter most.
python
import pdb; pdb.set_trace()def buggy_function():
a = 5
b = 0
c = a / b
Error here!
When the program hits `pdb.set_trace()`, it will pause execution, and you can step through the code line by line. This technique is invaluable when tracking down tricky bugs.
Remember, Python’s beauty lies in its simplicity, but that doesn’t mean it lacks depth. The more you explore, the more you'll discover how to make Python work for you, not the other way around.
all images in this post were generated using AI tools
Category:
ProgrammingAuthor:
Adeline Taylor
rate this article
18 comments
Jace McClure
This article offers invaluable insights for Python enthusiasts, blending practical tips with advanced techniques to enhance coding efficiency. From optimizing workflows to utilizing powerful libraries, the strategies presented can significantly elevate a programmer's skill set. Mastering these concepts will undoubtedly lead to cleaner, faster, and more efficient code. Great read!
April 6, 2025 at 3:33 AM
Adeline Taylor
Thank you for your feedback! I'm glad you found the insights helpful for enhancing coding efficiency. Happy coding!
Karson Jimenez
This article is a fantastic resource for anyone looking to enhance their Python skills. The tips are practical and easy to understand, making coding more efficient and enjoyable. I truly appreciate the effort put into sharing this knowledge with the community. Thank you for inspiring us to grow!
March 7, 2025 at 9:27 PM
Adeline Taylor
Thank you for your kind words! I'm glad you found the tips helpful and inspiring. Happy coding!
Spike McConnell
Great tips! Python's versatility is amazing, and these tricks will definitely help streamline my coding process. Excited to implement these techniques and boost my efficiency. Keep the awesome content coming!
February 18, 2025 at 7:48 PM
Adeline Taylor
Thank you! I'm glad you found the tips helpful. Excited to see how you implement them! Keep coding efficiently!
Francesca Matthews
Great article! These tips and tricks are invaluable for anyone looking to enhance their Python skills. I particularly loved the section on code optimization—it’s amazing how small changes can lead to big improvements in efficiency. Looking forward to trying these out in my next project!
February 4, 2025 at 12:48 PM
Adeline Taylor
Thank you for the kind words! I'm glad you found the tips helpful—good luck with your next project!
Ziva Whitley
Ready to turn your Python skills from 'meh' to 'wow'? Dive into these tips and tricks and watch your code dance! 🐍✨ Let's code efficiently and have fun doing it!
January 31, 2025 at 4:52 AM
Adeline Taylor
Thanks for your enthusiasm! Let's get coding and make Python fun and efficient together! 🐍✨
Rivenheart McGeehan
Great insights! I'm eager to explore these tips further. It's fascinating how small tweaks can lead to more efficient coding. Excited to enhance my Python skills!
January 30, 2025 at 9:26 PM
Adeline Taylor
Thank you! I'm glad you found the tips helpful. Happy coding and enjoy your journey to mastering Python!
Leona McDowney
Mastering Python is like learning to dance; it takes practice, a good rhythm, and occasionally stepping on a few toes! With these tips, you’ll be doing the robot in no time!
January 30, 2025 at 4:51 AM
Adeline Taylor
Absolutely! Just like dancing, mastering Python requires patience and a willingness to learn from mistakes. Keep practicing, and you'll soon find your groove!
Thistle Henderson
Great article! Mastering Python is essential for both beginners and seasoned programmers. I love the practical tips you've shared—especially the focus on code efficiency. These insights will undoubtedly help many of us streamline our coding practices. Thanks for sharing your expertise! Keep it up!
January 29, 2025 at 12:41 PM
Adeline Taylor
Thank you for your kind words! I'm glad you found the tips helpful. Happy coding!
Roxanne Ward
In the labyrinth of Python coding, secrets lie just beneath the surface. Unraveling its intricacies can lead to a mastery that transforms your projects. Dive deep, embrace these tips, and unlock the hidden potential waiting to be discovered. What mysteries will you unveil?
January 28, 2025 at 4:20 AM
Adeline Taylor
Thank you for your insightful comment! Indeed, diving deep into Python’s nuances can reveal powerful efficiencies in our coding journey. Happy coding!
Emmett McAndrews
Great insights! These tips will definitely enhance coding efficiency and streamline the learning process in Python.
January 27, 2025 at 9:03 PM
Adeline Taylor
Thank you! I'm glad you found the tips helpful for enhancing your Python coding journey!
Sable Clark
Essential insights for elevating your Python skills!
January 26, 2025 at 12:51 PM
Adeline Taylor
Thank you! I'm glad you found the insights helpful for enhancing your Python skills!
Angie Johnson
Great tips! Incorporating these tricks can significantly enhance coding efficiency. Looking forward to implementing them in my projects!
January 26, 2025 at 3:28 AM
Adeline Taylor
Thank you! I'm glad you found the tips helpful. Good luck with your projects!
Cerys Nelson
Great insights! I love how mastering Python can unlock so many possibilities in coding. The tips and tricks you’ve shared seem like game-changers for improving efficiency. I’m excited to try them out and see how they enhance my projects. Looking forward to more articles like this!
January 25, 2025 at 9:38 PM
Adeline Taylor
Thank you for your kind words! I’m glad you found the tips helpful, and I can't wait to hear how they enhance your projects. Stay tuned for more insights!
Drake Dodson
Great insights! These tips will definitely enhance productivity and streamline Python coding practices. Thanks!
January 25, 2025 at 11:22 AM
Adeline Taylor
Thank you! I'm glad you found the tips helpful. Happy coding!
Natalie Kane
Great insights! Embrace these tips to boost your Python skills and confidence!
January 24, 2025 at 8:17 PM
Adeline Taylor
Thank you! I’m glad you found the insights helpful for enhancing your Python skills!
Esme Rhodes
Great tips! Because nothing says 'mastering' like Googling how to use a library!
January 23, 2025 at 3:49 AM
Adeline Taylor
Thank you! Google is a powerful tool for learning and mastering new skills—it's all part of the journey!
Sierra Frank
Great article! It’s inspiring to see practical tips for mastering Python. Learning programming can be challenging, but your insights make it more approachable. Remember, everyone learns at their own pace, so be kind to yourself during the process. Happy coding, and keep up the great work!
January 22, 2025 at 2:01 PM
Adeline Taylor
Thank you so much for your kind words! I'm glad you found the tips helpful. Happy coding!
Holly McClain
Great insights on Python! The tips are practical and will definitely enhance coding efficiency for developers.
January 21, 2025 at 7:57 PM
Adeline Taylor
Thank you! I'm glad you found the tips helpful for enhancing coding efficiency in Python. Happy coding!
Exploring the World of Game Development with Unity
Why USB Hubs are Essential for Expanding Your Device’s Connectivity
Advanced Algorithms: Optimizing Performance in Large-Scale Systems
The Future of Smart Watches: Health Beyond the Wrist
Voice vs. Text: Interacting with Your Digital Assistant More Efficiently
How to Capture Dynamic Footage with FPV Drones
Applications of Quantum Computing in Healthcare and Medicine
Tools Every Content Creator Should Have in Their Toolkit
Autonomous Drones: The Future of Delivery and Beyond
The Impact of Low-Code Platforms on the Developer Community
The Hidden Security Challenges of 5G Networks